In this article, I’m explaining about a Split () method of
String class in C#.
Split:
Here into the ASP.Net C#, Split string function provides the functionality to split the string into a string array by specifying its delimiters.
The C# split string function splits the string into array collection according to the number of separators passed to the split function.
Where ASP.Net C# split string function removes the delimiters from the string and stores each part separated at consecutive indexes of an array object.
According to ASP.Net 2.0, C# split string function has 6 overloads as follows.
Split Function is overloaded
- The public string [] Split (char [] separator)
- The public string [] Split (char [] separator, int count)
- The public string [] Split (char [] separator, StringSplitOptions options)
- The public string [] Split (string [] separator, StringSplitOptions options)
- The public string [] Split (char [] separator, int count, StringSplitOptions options)
- The public string [] Split (string [] separator, int count, StringSplitOptions options)
Example:
Public string [] Split (char [] separator)
The following example demonstrates how to extract individual words from a block of text by treating white space and punctuation marks as delimiters.
The character array passed to the separator parameter of the String. Split (Char[]) method consists of a space character and a tab character, together with some common punctuation symbols.
namespace Split
{
class Program
{
static void Main(string[] args)
{
string words = "a,b,c,d,e,f,g,h,I,j,k,l,m";
string[] split = words.Split(new char[]{','});
foreach (string word in split)
{
Console.Write(word+" ");
}
Console.ReadLine();
}
}
}
Output
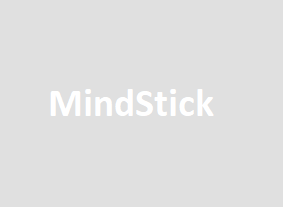
public string [] Split (char [] separator, int count)
This example demonstrates how to count affects the number of the string returned by Split.
namespace split2
{
class Program
{
public static void Main()
{
string words = "a,b,c,d,e,f,g,h,i,j";
string[] split = words.Split(new char[] { ',' }, 3);
foreach (string word in split)
{
Console.WriteLine(word);
}
Console.ReadLine(); }
}
}
The above example returned three substrings as specified in the count parameter of the C# split string function.
It returned first and the second substring separated from the specified delimiter and third substring unchanged.
Output
Public string [] Split (char [] separator, StringSplitOptions options)
The following example uses the StringSplitOptions enumeration to include or exclude substrings generated by the Split () method.
namespace Split3
{
class Program
{
public static void Main()
{
string s1 = ",ONE,,TWO,,,THREE,,";
char[] delimiter = new char[] { ',' };
string[] stringSeparators = new string[] { "[stop]" };
string[] result;
Console.WriteLine("1)The original string is. {0}", s1);
Console.WriteLine("The delimiter character is. '{0}'", delimiter[0]);
result = s1.Split(delimiter, StringSplitOptions.None);
Show(result);
Console.WriteLine("1) Split a string delimited by characters and " +"return all non-empty elements:");
result = s1.Split(delimiter, StringSplitOptions.RemoveEmptyEntries);
Show(result);
}
public static void Show(string[] entries)
{
Console.WriteLine("The return value contains these {0} elements:", entries.Length);
foreach (string entry in entries)
{
Console.Write("<{0}>", entry);
}
Console.ReadLine();
}
}
}
Output
Public string [] Split (string [] separator, StringSplitOptions options)
The following example defines an array of separator that includes punctuation and white-space characters.
Passing this array along with a value of StringSplitOptions.RemoveEmptyEntries to the Split (String [], StringSplitOptions) method returns an array that consists of the individual words from the string.
namespace Split4
{
class Program
{
static void Main(string[] args)
{
string[] separators = { ",", ".", "!", "?", ";", ":", " " };
string value = "MindStick,Software!pvt.?ltd..:";
string[] words = value.Split(separators, StringSplitOptions.RemoveEmptyEntries);
foreach (var word in words)
Console.Write(word+" ");
Console.ReadLine();
}
}
}
Output
public string [] Split (char [] separator, int count, StringSplitOptions options)
namespace Split5
{
class Program
{
static void Main(string[] args)
{
string s1 = ",ONE,,TWO,,,THREE,,";
char[] delimiter = new char[] { ',' };
string[] stringSeparators = newstring[] { "[stop]" };
string[] result;
Console.WriteLine("1)The original string is. {0}", s1);
Console.WriteLine("The delimiter character is. '{0}'", delimiter[0]);
result = s1.Split(delimiter,3, StringSplitOptions.None);
Show(result);
Console.WriteLine("1) Split a string delimited by characters and " + "return all non-empty elements:");
result = s1.Split(delimiter,2, StringSplitOptions.RemoveEmptyEntries);
Show(result);
}
publicstaticvoid Show(string[] entries)
{
Console.WriteLine("The return value contains these {0} elements:", entries.Length);
foreach (string entry in entries)
{
Console.Write("<{0}>", entry);
}
Console.ReadLine();
}
}
}
Output
Into my next post, I'll discuss about simple tooltip using HTML CSS
Leave Comment