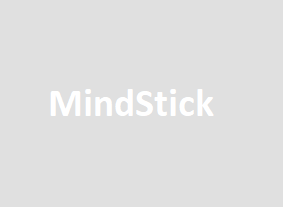
class subclass_name : access_mode base_class
{
//body of subclass
};
EXAMPLE:
// C++ program to explain
// Single inheritance
#include <iostream>
using namespace std;
// base class
class Vehicle {
public:
Vehicle()
{
cout << "This is a Vehicle" << endl;
}
};
// sub class derived from two base classes
class Car: public Vehicle{
};
// main function
int main()
{
// creating object of sub class will
// invoke the constructor of base classes
Car obj;
return 0;
}
Output:
This is a vehicle
2) MULTIPLE INHERITANCE:
In multiple inheritance properties of sub/chlid class are derived from from two super/parent classes.
EXAMPLE:
// C++ program to explain
// multiple inheritance
#include <iostream>
using namespace std;
// first base class
class Vehicle {
public:
Vehicle()
{
cout << "This is a Vehicle" << endl;
}
};
// second base class
class FourWheeler {
public:
FourWheeler()
{
cout << "This is a 4 wheeler Vehicle" << endl;
}
};
// sub class derived from two base classes
class Car: public Vehicle, public FourWheeler {
};
// main function
int main()
{
// creating object of sub class will
// invoke the constructor of base classes
Car obj;
return 0;
}
Output:
This is a Vehicle
This is a 4 wheeler Vehicle
3) MULTILEVEL INHERITANCE:
In multilevel inheritance,a derived class is derived from another base class.
EXAMPLE:
// C++ program to implement
// Multilevel Inheritance #include <iostream>
using namespace std;
// base class
class Vehicle
{
public:
Vehicle()
{
cout << "This is a Vehicle" << endl;
}
};
class fourWheeler: public Vehicle
{ public:
fourWheeler()
{
cout<<"Objects with 4 wheels are vehicles"<<endl;
} };
// sub class derived from two base classes
class Car: public fourWheeler{
public:
car()
{
cout<<"Car has 4 Wheels"<<endl;
}
};
// main function
int main()
{
//creating object of sub class will
//invoke the constructor of base classes
Car obj;
return 0;
}
output:
This is a Vehicle
Objects with 4 wheels are vehicles
Car has 4 Wheels
4) HIERARCHICAL INHERITANCE:
In hierarchical inheritance,more than one sub class is inherited from a single base class. i.e. more than one derived class is created from a single base class.
EXAMPLE:
// C++ program to implement
// Hierarchical Inheritance
#include <iostream>
using namespace std;
// base class
class Vehicle
{
public:
Vehicle()
{
cout << "This is a Vehicle" << endl;
}
};
// first sub class
class Car: public Vehicle {
};
// second sub class
class Bus: public Vehicle
{
};
// main function
int main()
{
// creating object of sub class will
// invoke the constructor of base class
Car obj1;
Bus obj2;
return 0;
}
Output:
This is a Vehicle
This is a Vehicle
5) HYBRID INHERITANCE:
Hybrid Inheritance is implemented by combining more than one type of inheritance. For example: Combining Hierarchical inheritance and Multiple Inheritance.
EXAMPLE:
// C++ program for Hybrid Inheritance
#include <iostream>
using namespace std;
// base class
class Vehicle {
public: Vehicle()
{
cout << "This is a Vehicle" << endl; } };
//base class
class Fare
{
public:
Fare()
{
cout<<"Fare of Vehicle\n";
}
};
// first sub class
class Car: public Vehicle
{
};
// second sub class
class Bus: public Vehicle, public Fare
{
};
// main function
int main()
{
// creating object of sub class will
// invoke the constructor of base class
Bus obj2;
return 0;
}
Output:
This is a Vehicle
Fare of Vehicle
TYPES OF INHERITANCE SUPPORTED BY JAVA:
Three types of inheritance are supported by java which are:
1)Single Inheritance
2)Multilevel Inheritance
3)Hierarchical Inheritance
There are two more types of inheritance supported by java but through interface only:
1)Multiple Inheritance
2)Hybrid Inheritance
The reason that Java does not support multiple inheritances is that of the problem known as "diamond problem".
Leave Comment