Forms and validation are crucial aspects of any web application, and AngularJS provides robust support for handling both. Here's a detailed guide on how to manage forms and validation in AngularJS.
I hope, You know about the HTML form and its uses. There are some changes in your code for angular js.
Create a form with validations –
1. Creating a Form AngularJS provides the ng-form directive to create forms. Here's a basic example:
<div class="container" ng-app="myApp" ng-controller="MySimpleController">
<h2>AngularJS Form</h2>
<hr>
<!-- Create a form -->
<form name="userForm" class="row g-3" accept="" method="" novalidate>
<!-- Create a field for name model -->
<div class="col-12">
<label class="form-label fw-bold">Name</label>
<input type="text" ng-model="user.name" class="form-control" placeholder="Name"
autofocus="true" required />
</div>
<!-- Create a filed for email model -->
<div class="col-12">
<label class="form-label fw-bold">Email</label>
<input type="email" ng-model="user.email" class="form-control" placeholder="Email"
autofocus="true" required />
</div>
<!-- Create a field for age model -->
<div class="col-12">
<label class="form-label fw-bold">Age</label>
<input type="number" ng-model="user.age" class="form-control" placeholder="Age"
autofocus="true" required />
</div>
<!-- Create a field for about model -->
<div class="col-12">
<label class="form-label fw-bold">About</label>
<textarea type="text" ng-model="user.about" class="form-control" placeholder="About"
autofocus="true" required></textarea>
</div>
<div class="col-12">
<button class="btn btn-primary" ng-disabled="userForm.$invalid">Submit</button>
<div>
</form>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('MySimpleController', function ($scope) {
$scope.user = { name: '', email: '', age: null, about: '' };
});
</script>
2. Adding Validation AngularJS provides built-in validation directives that you can use directly on form elements:
- required: Ensures the field is not empty.
- ng-minlength and ng-maxlength: Specifies minimum and maximum length for input.
- ng-pattern: Validates the input based on a regular expression.
- type="email": Checks if the input is a valid email.
- type="number": Ensures the input is a number.
Here’s an extended version of the previous example with additional validations:
<div class="container" ng-app="myApp" ng-controller="MySimpleController">
<h2>AngularJS Form</h2>
<hr>
<!-- Create a form -->
<form name="userForm" class="row g-3" accept="" method="" novalidate>
<!-- Create a field for name model -->
<div class="col-12">
<label class="form-label fw-bold">Name</label>
<input type="text" id="name" name="name" ng-model="user.name" ng-minlength="3" ng-maxlength="20"
class="form-control" placeholder="Name" autofocus="true" required />
<p class="text-danger">
<span ng-show="userForm.name.$error.required && userForm.name.$touched">Name is required.</span>
<span ng-show="userForm.name.$error.minlength">Name is too short.</span>
<span ng-show="userForm.name.$error.maxlength">Name is too long.</span>
</p>
</div>
<!-- Create a filed for email model -->
<div class="col-12">
<label class="form-label fw-bold">Email</label>
<input type="email" ng-model="user.email" class="form-control" placeholder="Email" autofocus="true"
required />
<p class="text-danger">
<span ng-show="userForm.email.$error.required && userForm.email.$touched">Email is required.</span>
<span ng-show="userForm.email.$error.email">Invalid email address.</span>
</p>
</div>
<!-- Create a field for age model -->
<div class="col-12">
<label class="form-label fw-bold">Age</label>
<input type="number" id="age" name="age" ng-model="user.age" min="0" max="120" class="form-control"
placeholder="Age" autofocus="true" required />
<p class="text-danger">
<span ng-show="userForm.age.$error.required && userForm.age.$touched">Age is required.</span>
<span ng-show="userForm.age.$error.min">Age must be at least 0.</span>
<span ng-show="userForm.age.$error.max">Age must be at most 120.</span>
</p>
</div>
<!-- Create a field for about model -->
<div class="col-12">
<label class="form-label fw-bold">About</label>
<textarea type="text" id="about" name="about" ng-model="user.about" class="form-control"
placeholder="About" autofocus="true" required></textarea>
<p class="text-danger">
<span ng-show="userForm.about.$error.required && userForm.about.$touched">About is required.</span>
</p>
</div>
<div class="col-12">
<button class="btn btn-primary" ng-disabled="userForm.$invalid">Submit</button>
<div>
</form>
</div>
3. Form State and Validation Classes
AngularJS provides several CSS classes that indicate the state of the form and its fields:
- ng-valid / ng-invalid: The field is valid/invalid.
- ng-pristine / ng-dirty: The field has not been modified/modified.
- ng-touched / ng-untouched: The field has been blurred/never blurred.
You can use these classes to style the form elements based on their state.
<style>
.ng-invalid.ng-touched {
border-color: red;
}
.ng-valid.ng-touched {
border-color: green;
}
</style>
5. Handling Form Submission
When handling form submissions, you typically want to process the data only if the form is valid. You can do this by checking the $valid property of the form.
<div class="container" ng-app="myApp" ng-controller="MySimpleController">
<h2>AngularJS Form</h2>
<hr>
<!-- Create a form -->
<form name="userForm" class="row g-3" accept="" method="" ng-submit="submitForm(userForm.$valid)" novalidate>
<!-- Create a field for name model -->
<div class="col-12">
<label class="form-label fw-bold">Name</label>
<input type="text" id="name" name="name" ng-model="user.name" ng-minlength="3" ng-maxlength="20"
class="form-control" placeholder="Name" autofocus="true" ng-pattern="/^[a-zA-Z0-9._-]+$/" required />
<p class="text-danger">
<span ng-show="userForm.name.$error.required && userForm.name.$touched">Name is required.</span>
<span ng-show="userForm.name.$error.minlength">Name is too short.</span>
<span ng-show="userForm.name.$error.maxlength">Name is too long.</span>
<span ng-show="userForm.name.$error.pattern">Invalid name format.</span>
</p>
</div>
<!-- Create a filed for email model -->
<div class="col-12">
<label class="form-label fw-bold">Email</label>
<input type="email" ng-model="user.email" class="form-control" placeholder="Email" autofocus="true"
required />
<p class="text-danger">
<span ng-show="userForm.email.$error.required && userForm.email.$touched">Email is required.</span>
<span ng-show="userForm.email.$error.email">Invalid email address.</span>
</p>
</div>
<!-- Create a field for age model -->
<div class="col-12">
<label class="form-label fw-bold">Age</label>
<input type="number" id="age" name="age" ng-model="user.age" min="0" max="120" class="form-control"
placeholder="Age" autofocus="true" required />
<p class="text-danger">
<span ng-show="userForm.age.$error.required && userForm.age.$touched">Age is required.</span>
<span ng-show="userForm.age.$error.min">Age must be at least 0.</span>
<span ng-show="userForm.age.$error.max">Age must be at most 120.</span>
</p>
</div>
<!-- Create a field for about model -->
<div class="col-12">
<label class="form-label fw-bold">About</label>
<textarea type="text" id="about" name="about" ng-model="user.about" class="form-control"
placeholder="About" autofocus="true" required></textarea>
<p class="text-danger">
<span ng-show="userForm.about.$error.required && userForm.about.$touched">About is required.</span>
</p>
</div>
<div class="col-12">
<button class="btn btn-primary" ng-disabled="userForm.$invalid">Submit</button>
<div>
</form>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('MySimpleController', function ($scope) {
$scope.user = { name: '', email: '', age: null, about: null };
$scope.checkUsername = function (value) {
// Custom validation logic
if (value) {
var isValid = value.match(/^[a-zA-Z0-9._-]+$/);
return isValid ? true : false;
}
return false;
};
$scope.submitForm = function (isValid) {
if (isValid) {
// Process form data
alert('Form is valid!');
} else {
alert('Form is invalid!');
}
};
});
</script>
5. Now add all code in one project
<!doctype html>
<html lang="en" data-ng-app="myApp">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>AngularJS Todo List</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH" crossorigin="anonymous">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.11.3/font/bootstrap-icons.min.css">
<!-- Angurr JS CDN -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.8.3/angular.min.js"
integrity="sha512-KZmyTq3PLx9EZl0RHShHQuXtrvdJ+m35tuOiwlcZfs/rE7NZv29ygNA8SFCkMXTnYZQK2OX0Gm2qKGfvWEtRXA=="
crossorigin="anonymous" referrerpolicy="no-referrer">
</script>
<style>
.ng-invalid.ng-touched {
border-color: red;
}
.ng-valid.ng-touched {
border-color: green;
}
</style>
</head>
<body>
<div class="container" ng-controller="MySimpleController as sip">
<h2>AngularJS Form</h2>
<hr />
<div>
<!-- Create a form -->
<form name="userForm" class="row g-3" accept="" method="" ng-submit="submitForm(userForm.$valid)"
novalidate>
<!-- Hidden fields -->
<input type="hidden" ng-model="userPost.id" />
<!-- Create a field for userId model -->
<div class="col-12">
<label class="form-label fw-bold">UserId</label>
<input type="number" id="userId" name="userId" ng-model="userPost.userId" class="form-control"
placeholder="userId" autofocus="true" required />
<p class="text-danger">
<span ng-show="userForm.userId.$error.required && userForm.userId.$touched">UserId is
required.</span>
</p>
</div>
<!-- Create a field for name model -->
<div class="col-12">
<label class="form-label fw-bold">Title</label>
<input type="text" id="title" name="title" ng-model="userPost.title" ng-minlength="3"
ng-maxlength="100" class="form-control" placeholder="Title" autofocus="true"
ng-pattern="/^[a-zA-Z0-9._-\s]+$/" required />
<p class="text-danger">
<span ng-show="userForm.title.$error.required && userForm.title.$touched">Title is
required.</span>
<span ng-show="userForm.title.$error.minlength">Title is too short.</span>
<span ng-show="userForm.title.$error.maxlength">Title is too long.</span>
<span ng-show="userForm.title.$error.pattern">Invalid title format.</span>
</p>
</div>
<!-- Create a field for about model -->
<div class="col-12">
<label class="form-label fw-bold">Body</label>
<textarea type="text" id="body" name="body" ng-model="userPost.body" class="form-control"
placeholder="Body" autofocus="true" required></textarea>
<p class="text-danger">
<span ng-show="userForm.body.$error.required && userForm.body.$touched">Body is required.</span>
</p>
</div>
<div class="col-12">
<div class="d-flex justify-content-between flex-column flex-md-row">
<div>
<button class="btn btn-secondary" type="button" data-ng-click="resetForm()">Reset</button>
<button class="btn btn-primary" type="submit"
ng-disabled="userForm.$invalid">Submit</button>
</div>
<div class="d-flex flex-column-reverse flex-md-row align-items-center">
<p class="fw-bold px-2 m-0 my-1 my-md-0"
data-ng-bind="filteredPosts.length + ' record found'"></p>
<input type="search" data-ng-model="searchText" class="form-control mt-2 mt-md-0 w-auto"
placeholder="Search ..." />
</div>
</div>
</div>
</form>
</div>
<hr>
<div class="row row-cols-1 row-cols-md-3 g-3 mb-3">
<div class="w-100 py-3"
data-ng-if="(filteredPosts = (Posts | orderBy:'id':true | customFilter:searchText )).length == 0">
<div class="d-flex justify-content-center fw-bold">
<div class="text-center">
<p class=" fs-2 ">
<span class="me-2">😕</span>
No records were found
</p>
<p class="fw-normal">try somthing new keywords</p>
</div>
</div>
</div>
<div class="col" data-ng-repeat="post in filteredPosts">
<div class="card h-100">
<div class="card-header bg-transparent border-success position-relative">
<span class="px-2 position-absolute end-0 me-2 " data-post-id="{{post.id}}">
<button class="btn btn-link p-0" title="Edit" data-ng-click="editPost(post.id)"> <span
class="bi bi-pencil me-1"></span></button>
<button class="btn btn-link p-0" title="Delete" data-ng-click="deletePost(post.id)"> <span
class="bi bi-trash me-1 text-danger"></span></button>
</span>
<p class=" fw-bold m-0">
<span class="bi bi-person me-1"></span>
{{post.id}}
</p>
</div>
<div class="card-body">
<h5 class="card-title">
{{post.title}}
</h5>
<p class="mb-1">
{{post.body}}
</p>
</div>
</div>
</div>
</div>
</div>
<script>
var app = angular.module('myApp', []);
// create custom search filter like type any keyword in search box and display in the ui format
app.filter('customFilter', function () {
return function (items, searchText) {
if (!searchText) {
return items;
}
searchText = searchText.toLowerCase();
return items.filter(function (item) {
return Object.keys(item).some(function (key) {
if (typeof item[key] === 'object') {
return Object.keys(item[key]).some(function (subKey) {
return String(item[key][subKey]).toLowerCase().includes(searchText);
});
}
return String(item[key]).toLowerCase().includes(searchText);
});
});
};
});
app.controller('MySimpleController', ['$scope', function ($scope) {
$scope.Posts = [{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
},
{
"userId": 1,
"id": 2,
"title": "qui est esse",
"body": "est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla" }, { "userId": 1, "id": 3, "title": "ea molestias quasi exercitationem repellat qui ipsa sit aut", "body": "et iusto sed quo iure\nvoluptatem occaecati omnis eligendi aut ad\nvoluptatem doloribus vel accusantium quis pariatur\nmolestiae porro eius odio et labore et velit aut" }, { "userId": 1, "id": 4, "title": "eum et est occaecati", "body": "ullam et saepe reiciendis voluptatem adipisci\nsit amet autem assumenda provident rerum culpa\nquis hic commodi nesciunt rem tenetur doloremque ipsam iure\nquis sunt voluptatem rerum illo velit" }, { "userId": 1, "id": 5, "title": "nesciunt quas odio", "body": "repudiandae veniam quaerat sunt sed\nalias aut fugiat sit autem sed est\nvoluptatem omnis possimus esse voluptatibus quis\nest aut tenetur dolor neque" }, { "userId": 1, "id": 6, "title": "dolorem eum magni eos aperiam quia", "body": "ut aspernatur corporis harum nihil quis provident sequi\nmollitia nobis aliquid molestiae\nperspiciatis et ea nemo ab reprehenderit accusantium quas\nvoluptate dolores velit et doloremque molestiae" }, { "userId": 1, "id": 7, "title": "magnam facilis autem", "body": "dolore placeat quibusdam ea quo vitae\nmagni quis enim qui quis quo nemo aut saepe\nquidem repellat excepturi ut quia\nsunt ut sequi eos ea sed quas" }, { "userId": 1, "id": 8, "title": "dolorem dolore est ipsam", "body": "dignissimos aperiam dolorem qui eum\nfacilis quibusdam animi sint suscipit qui sint possimus cum\nquaerat magni maiores excepturi\nipsam ut commodi dolor voluptatum modi aut vitae" }, { "userId": 1, "id": 9, "title": "nesciunt iure omnis dolorem tempora et accusantium", "body": "consectetur animi nesciunt iure dolore\nenim quia ad\nveniam autem ut quam aut nobis\net est aut quod aut provident voluptas autem voluptas" }, { "userId": 1, "id": 10, "title": "optio molestias id quia eum", "body": "quo et expedita modi cum officia vel magni\ndoloribus qui repudiandae\nvero nisi sit\nquos veniam quod sed accusamus veritatis error" }]; $scope.userPostMaster = { title: '', userId: 10, id: 0, body: null }; $scope.userPost = angular.copy($scope.userPostMaster); $scope.getPostById = function (postid) { const index = $scope.Posts.findIndex(p => p.id === postid); if (index !== -1) { return $scope.Posts[index]; } } $scope.getLastPost = function () { if (!$scope.Posts || $scope.Posts.length === 0) { return null; } return $scope.Posts[$scope.Posts.length - 1]; }; // Function to submit the form $scope.submitForm = function (isValid) { if (isValid) { if ($scope.userPost.id) { // Find the index of the updated post const index = $scope.Posts.findIndex(p => p.id === $scope.userPost.id); if (index !== -1) { // Update the post in the array $scope.Posts[index] = angular.copy($scope.userPost); } alert('Post updated successfully!'); } else { // Handle the response after successful submission if ($scope.getLastPost() != null) { var newPost = angular.copy($scope.userPost); //console.log(newPost, $scope.getLastPost()); newPost.id = $scope.getLastPost().id + 1; $scope.Posts.push(newPost); } else { $scope.userPost.id = 1; $scope.Posts.push($scope.userPost); } alert('Form submitted successfully!'); } $scope.resetForm(); } else { alert('Form is invalid!'); } }; // Function to delete a post $scope.deletePost = function (postId) { if (window.confirm("Are you sure, You want to delete this record.")) { // On successful deletion, remove the post from the scope const index = $scope.Posts.findIndex(p => p.id === postId); if (index !== -1) { // Update the post in the array $scope.Posts.splice(index, 1) } alert('Post deleted successfully!'); } }; // Function to fetch a post by ID for editing $scope.editPost = function (postId) { $scope.userPost = angular.copy($scope.getPostById(postId)); }; // Function to reset the form $scope.resetForm = function () { // Reset the post model $scope.userPost = angular.copy($scope.userPostMaster); // Reset form state $scope.userForm.$setPristine(); $scope.userForm.$setUntouched(); }; }]); </script> </body> </html>
Output -
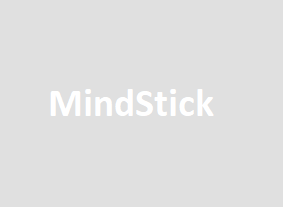
Thank you.
Leave Comment