RESTFul APIs in AngularJS
AngularJS uses AngularJS’s built-in services to make HTTP
requests to retrieve data from RESTful APIs. Here is a step-by-step guide to fetch data from a RESTful API in AngularJS,
Set Up Your AngularJS Application
First, make sure your project includes AngularJS. You can download or use the CDN link in your HTML file.
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
<script src="app.js"></script> <!-- Your AngularJS application script -->
</head>
<body>
<div ng-controller="MainController">
<!-- Display fetched data here -->
</div>
</body>
</html>
Create your AngularJS Module and Controller
Define your AngularJS module and controller. Load the $http
service, which is used to make HTTP requests.
// app.js
var app = angular.module('myApp', []);
// add angular services in controller
app.controller('MainController', ['$scope', '$http', function($scope, $http) {
// Function to fetch data
$scope.fetchData = function() {
$http.get('https://api.example.com/data')
.then(function(response) {
// Handle successful response
$scope.data = response.data;
}, function(error) {
// Handle error
console.error('Error fetching data:', error);
});
};
// Initial fetch when controller loads
$scope.fetchData();
}]);
The other way to fetch data from RESTful API using $http({…})
method is as follow,
angular.module('myApp', [])
.controller('MyController', function ($scope, $http) {
// fetch data from API
$http({
url: "https://api.example.com/data",
method : "GET",
DataType: "json"
}).then(function(response){
$scope.items = response.data;
}).catch(function(error){
console.log("error: ", error);
});
});
Fetch Data Using $http
In the controller, use the $http.get
method to retrieve data from your RESTful API. Replace 'https://api.example.com/data' with your actual API endpoint.
success Handler- .then (function(response) { ... })
- This function is called if the HTTP request is successful. response.data contains the response data from the API.
- Assign the fetched data to a
$scope
variable (e.g.,$scope.data
) and use it in your HTML.
Error handler- .catch(function(error) { ... }) .
- This function handles any errors that occur during an HTTP request.
Show Fetched Data in your HTML
Update your HTML to display data fetched with AngularJS directives.
<div ng-controller="MainController">
<ul>
<li ng-repeat="item in data">{{ item.name }} - {{ item.description }}</li>
</ul>
</div>
In the example above
ng-controller="MainController"
Assigns the controller to a specific part of the HTML.ng-repeat="object in data"
Repeats over each object in the data structure retrieved from the API.{{ item.name }}
and{{ item.description }}
specify the attributes of each item in the list.
Example-
<!DOCTYPE html>
<html data-ng-app="myApp" lang="en">
<head>
<meta charset="UTF-8">
<title>AngularJS Loop Example</title>
<!-- bootstrap cdn link -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- angular js cdn link -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
</head>
<body>
<div data-ng-controller="MyController" class="container my-4">
<!-- bind the controller data into bootstrap card -->
<div class="row row-cols-1 row-cols-md-3 g-4">
<div data-ng-repeat="data in items" class="col">
<div class="card h-100">
<div class="card-header">
<span class="p-1 rounded-circle bg-primary-subtle">{{data.id}}</span>
</div>
<div class="card-body">
<p class="card-title fw-semibold m-0">{{data.title}}</p>
<p class="card-text">{{data.body}}</p>
</div>
</div>
</div>
</div>
</div>
<script>
angular.module('myApp', [])
.controller('MyController', function ($scope, $http) {
console.log("hello");
// fetch data from API
$http.get("https://jsonplaceholder.typicode.com/posts")
.then(function (response) {
// take first 15 records only
$scope.items = response.data.slice(0, 14);
console.log("data fetched");
// execute if any error occured
}).catch(function (error) {
console.log("error: ", error);
});
});
</script>
</body>
</html>
Output-
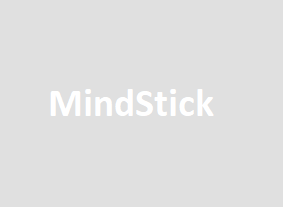
By following these steps, you can successfully fetch data from RESTful APIs in AngularJS and insert it into your web application.
Also, Read: Explain Routing in AngularJS
Leave Comment