AngularJS Single Page Application
Building a Single Page Application (SPA) in AngularJS requires you to configure your application to load all the necessary code (HTML, CSS, JavaScript) into the first single page. AngularJS makes this easy by allowing you to define methods, templates, and controllers, and makes it easy to move between different views without having to refresh the entire page.
Here's a step-by-step guide to creating a simple SPA using AngularJS,
1. Configure your AngularJS application
First, add AngularJS to your HTML file. You can either download AngularJS or use the CDN link.
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<meta charset="utf-8">
<title>AngularJS SPA Example</title>
<!-- bootstrap cdn -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- Angular js cdn -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script>
<!-- Angular js routing cdn -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular-route.js"></script>
<!-- Your AngularJS application script -->
<script src="main.js"></script>
</head>
<body>
<!-- Placeholder for views -->
<div ng-view></div>
</body>
</html>
2. Define your AngularJS Module and configure Routing
In your main.js (or any JavaScript file), define your AngularJS module, configure routing using
$routeProvider
, and create controllers for each view.
// Routing code for load view page
.config(function($routeProvider){
$routeProvider
// home page
.when('/home', {
templateUrl: 'Home.html',
controller: 'homeController'
})
// load when click on userdata
.when('/userdata', {
templateUrl : 'ng-RouteUserData.html',
controller : 'userController'
})
// laod when click on posts
.when('/posts', {
templateUrl : 'ng-RoutePosts.html',
controller : 'postController'
})
// default load for ng-route.html
.otherwise({
redirectTo: '/'
});
})
// controllers of all pages
.controller("homeController", ["$scope", function($scope){
console.log("Home page");
}])
.controller('userController',['$scope', '$http', '$interval', function($scope, $http, $interval){
console.log("Home page");
}])
// Angular code for Posts.html page
.controller('postController',['$scope', '$http', '$interval', function($scope, $http, $interval){
console.log("Home page");
}]);
3. Create Your Views
Create separate HTML files for each view (home.html and about.html) in the view folder.
Home.html
<h1>{{ message }}</h1>
<p>This is the home page content.</p>
ng-RoutePosts.html
<h1>{{ message }}</h1>
<p>This is the Posts page content.</p>
ng-RouteUserData.html
<h1>{{ message }}</h1>
<p>This is the UserList page content.</p>
4. Set Up Navigation
Add navigation links (<a> tags) between views in your main HTML file.
<!-- ng-route.html -->
<body>
<div>
<a href="#/">Home</a>
<a href="#/about">About</a>
</div>
<div ng-view></div> <!-- Placeholder for views -->
</body>
In the example above
ng-app="myApp"
: Describes the AngularJS application module.
ngRoute
: AngularJS module routing.
$routeProvider
: Configures routes using the .when() and .otherwise() methods.
ng-view
: A directive in which AngularJS will display the views associated with each method.
Controllers
: Define controllers for each view to handle logic and data specific to that view.
Navigation: Use <a href="#/...">
to navigate between views. AngularJS will handle routing without full page reload.
Also, Read: Explain Routing in AngularJS
Example-
ng-route.html file (Index file)
<!DOCTYPE html>
<html data-ng-app="myApp">
<title>AngularJS Routing</title>
<!-- bootstrap cdn -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- Angular js cdn -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script>
<!-- Angular js routing cdn -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular-route.js"></script>
<body>
<div class="container my-4">
<div class="d-flex justify-content-between">
<p class="m-0">
<a ng-href="#!home/"
class="text-dark text-decoration-none fs-4 fw-bold bg-primary-subtle rounded-3 px-2 p-1">ngRoute</a>
</p>
<p class="ms-auto m-0 align-content-around">
<span>
<a ng-href="#!home/"
class="link-offset-2 link-offset-3-hover link-underline link-underline-opacity-0 link-underline-opacity-75-hover">Home</a>
<a ng-href="#!userdata"
class="link-offset-2 link-offset-3-hover link-underline link-underline-opacity-0 link-underline-opacity-75-hover mx-1">UserData</a>
<a ng-href="#!posts"
class="link-offset-2 link-offset-3-hover link-underline link-underline-opacity-0 link-underline-opacity-75-hover mx-1">Posts</a>
</span>
</p>
</div>
<hr class="m-1" />
<!-- loads and bind the html file using ngRoute -->
<div data-ng-view></div>
</div>
<!-- bind the javascript file -->
<script src="main.js"> </script>
</body>
</html>
Home.html
<div data-ng-controller="homeController">
<div class="row" >
<div class="col-12 my-4">
<div class="text-center my-5">
<p class="fs-1 fw-semibold p-2">Angular Routing Example</p>
<div>
<p class="fw-5 ">
Here you learn about AngularJs Routing in details. Routing refers to the mechanism that allows you to define navigation paths within your application and map them to specific views or components that should be displayed based on the URL.
</p>
</div>
</div>
</div>
<div class="row row-cols-1 row-cols-md-3 g-4 my-3 py-5 bg-secondary-subtle">
<div class="col">
<div class="card h-100">
<img src="Angulars.jpg" class="card-img-top" alt="angular-1">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.</p>
</div>
</div>
</div>
<div class="col">
<div class="card h-100">
<img src="Angulars.jpg" class="card-img-top" alt="angular-2">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This card has supporting text below as a natural lead-in to additional content.</p>
</div>
</div>
</div>
<div class="col">
<div class="card h-100">
<img src="Angulars.jpg" class="card-img-top" alt="angular-3">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.</p>
</div>
</div>
</div>
</div>
</div>
</div>
ng-RoutePosts.html
<div data-ng-controller="postController">
<div class="row" >
<div class="col-12">
<h2>All Posts</h2>
<hr />
<div class="row row-cols-1 row-cols-md-3 g-4">
<div class="col" data-ng-repeat="post in myData">
<div class="card h-100">
<div class="card-header">
<span class="fw-semibold p-1 px-2 bg-primary rounded-circle">{{post.id}}</span>
</div>
<div class="card-body">
<a ng-href="{{urlData + '/' + post.id}}" class="text-decoration-none"><p class="card-title fw-semibold lh-sm">{{post.title}}</p></a>
<p class="card-text">{{post.body}}</p>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
ng-RouteUserData.html
<div data-ng-controller="userController">
<div class="d-flex justify-content-between m-2">
<p class="fs-5 fw-bold m-0">All Users</p>
<p class="justify-content-end m-0">
<span class="fw-semibold">Current Time: {{currTime}}</span>
</p>
</div>
<div class="row">
<div class="col-12">
<div class="row row-cols-1 row-cols-md-3 g-4">
<div class="col" data-ng-repeat="user in Users">
<div class="card h-100">
<div class="card-header">
<span class="fw-semibold p-1 px-2 bg-primary rounded-circle">{{user.id}}</span>
<span class="fw-semibold mx-2">{{user.username}}</span>
</div>
<div class="card-body">
<p class="card-title fw-semibold lh-sm"><span class="fw-semibold">Name:</span> {{user.name}}</p>
<p class="card-text"><span class="fw-semibold">Email ID:</span> {{user.email}}</p>
<p class="card-text"><span class="fw-semibold">Phone No: </span>{{user.phone}}</p>
<p class="card-text"><span class="fw-semibold">Address: </span>{{user.address.street+' '+user.address.city+' - '+user.address.zipcode}}</p>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
main.js (Main JavaScript file)
angular.module("myApp", ["ngRoute"])
// Routing code for load view page
.config(function($routeProvider){
$routeProvider
// home page
.when('/home', {
templateUrl: 'Home.html',
controller: 'homeController'
})
// load when click on userdata
.when('/userdata', {
templateUrl : 'ng-RouteUserData.html',
controller : 'userController'
})
// laod when click on posts
.when('/posts', {
templateUrl : 'ng-RoutePosts.html',
controller : 'postController'
})
// default load for ng-route.html
.otherwise({
redirectTo: '/'
});
})
// controllers of all pages
.controller("homeController", ["$scope", function($scope){
console.log("Home page");
}])
.controller('userController',['$scope', '$http', '$interval', function($scope, $http, $interval){
$interval(function(){
$scope.currTime = new Date().toLocaleTimeString();
});
// Angular code for userData page
$scope.urlUser = "https://jsonplaceholder.typicode.com/users";
$http({
method: "GET",
url : $scope.urlUser
}).then(function(response){
$scope.Users = response.data;
}).catch(function(error){
console.log("Error: ", error);
});
}])
// Angular code for Posts.html page
.controller('postController',['$scope', '$http', '$interval', function($scope, $http, $interval){
$scope.currTime = new Date().toLocaleTimeString();
$interval(function(){
$scope.currTime = new Date().toLocaleTimeString()
}, 1000);
$scope.urlData = "https://jsonplaceholder.typicode.com/posts";
$http({
method : "GET",
url : $scope.urlData
}).then(function Success(respnose){
$scope.myData = respnose.data.slice(0, 20);
}).catch(function OnError(error){
console.log("Error : ", error);
});
}]);
Note- All above file keep in same folder or directory
Output-
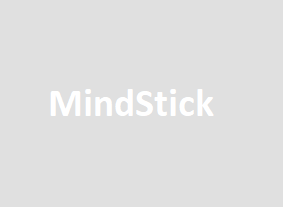
This template provides the basic framework for a Single Page Application in AngularJS. As your application grows, you can add more views, controllers, and services to handle complex use cases.
Also, Read: Fetch Data from RESTFul APIs in AngularJS
Leave Comment