Integrating KnockoutJS with other technologies like ASP.NET, Node.js, or RESTful APIs can significantly enhance the functionality and user experience of your web applications. Here’s a detailed guide on how to achieve this integration:
Integrating KnockoutJS with ASP.NET
Create an ASP.NET Project:
- Use Visual Studio to create a new ASP.NET Web Application.
- Choose the appropriate template (e.g., MVC or Web API).
Set Up KnockoutJS: Add KnockoutJS to your project via NuGet Package Manager or include it directly from a CDN in your HTML.
<!-- Link to Knockout.js library for MVVM pattern support -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.5.1/knockout-latest.min.js"
integrity="sha512-vs7+jbztHoMto5Yd/yinM4/y2DOkPLt0fATcN+j+G4ANY2z4faIzZIOMkpBmWdcxt+596FemCh9M18NUJTZwvw=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Creating the ViewModel: Define the ViewModel in JavaScript:
<script type="text/javascript">
function AppViewModel() {
var self = this;
self.items = ko.observableArray([]);
self.loadItems = function () {
$.getJSON('/api/Values', function (data) {
self.items(data);
console.log(data);
});
};
self.loadItems();
}
ko.applyBindings(new AppViewModel());
</script>
Create a Controller: In the Controllers folder, create a new controller that returns data.
using KnockoutJSAPI.Models;
using System;
using System.Collections.Generic;
using System.Web.Http;
using System.Linq;
namespace KnockoutJSAPI.Controllers
{
public class ValuesController : ApiController
{
private ICollection<Student> GetStudents()
{
// Create a list of students
ICollection<Student> students = new List<Student>
{
new Student
{
StudentId = 1,
FirstName = "John",
LastName = "Doe",
DOB = new DateTime(2000, 1, 1),
Email = "john.doe@example.com",
Phone = "123-456-7890",
FatherName = "Michael Doe",
MotherName = "Anna Doe",
Gender = "Male",
RollNo = 101,
ClassName = 10,
Section = 'A',
EnrollmentDate = DateTime.Now,
PermanentAddress = new Address
{
Street = "123 Main St",
City = "Springfield",
State = "IL",
PostalCode = "62701",
Country = "USA"
},
Photo = "https://www.gravatar.com/avatar/2c7d99fe281ecd3bcd65ab915bac6dd5?s=250",
LocalAddress = new Address
{
Street = "456 Elm St",
City = "Chicago",
State = "IL",
PostalCode = "60601",
Country = "USA"
},
Subjects = new List<Subject>
{
new Subject
{
SubjectId = 1,
SubjectName = "Mathematics",
Description = "Study of numbers and operations.",
Credits = 4
},
new Subject
{
SubjectId = 2,
SubjectName = "Science",
Description = "Study of the natural world.",
Credits = 3
}
}
},
// Add more students here...
new Student
{
StudentId = 2,
FirstName = "Jane",
LastName = "Smith",
DOB = new DateTime(2001, 3, 15),
Email = "jane.smith@example.com",
Phone = "987-654-3210",
FatherName = "David Smith",
MotherName = "Emily Smith",
Gender = "Female",
RollNo = 102,
ClassName = 10,
Section = 'B',
EnrollmentDate = DateTime.Now,
PermanentAddress = new Address
{
Street = "789 Oak Ave",
City = "Springfield",
State = "IL",
PostalCode = "62702",
Country = "USA"
},
Photo = "https://file.xunruicms.com/admin_html/assets/pages/media/profile/profile_user.jpg",
LocalAddress = new Address
{
Street = "567 Maple Ln",
City = "Chicago",
State = "IL",
PostalCode = "60602",
Country = "USA"
},
Subjects = new List<Subject>
{
new Subject
{
SubjectId = 3,
SubjectName = "English",
Description = "Study of language and literature.",
Credits = 3
},
new Subject
{
SubjectId = 4,
SubjectName = "History",
Description = "Study of past events.",
Credits = 2
}
}
}
// Add more students as needed...
};
return students;
}
// GET api/values
public IHttpActionResult Get()
{
return Ok(GetStudents());
}
// GET api/values/5
public IHttpActionResult Get(int StudentId)
{
return Ok(GetStudents().FirstOrDefault(x => x.StudentId == StudentId));
}
// POST api/values
public void Post([FromBody] string value)
{
}
// PUT api/values/5
public void Put(int id, [FromBody] string value)
{
}
// DELETE api/values/5
public void Delete(int id)
{
}
}
}
The Student
model inherited from different models like Person
,
Address
and Subject
models.
Person.cs
using System;
namespace KnockoutJSAPI.Models
{
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string FullName
{
get
{
return this.FirstName + " " + this.LastName;
}
}
public string Email { get; set; }
public string Phone { get; set; }
public string FatherName { get; set; }
public string MotherName { get; set; }
public string Gender { get; set; }
public Nullable<DateTime> DOB { get; set; }
public string Photo { get; set; }
public int Age
{
get
{
return (int)(!DOB.HasValue ? 0 : DateTime.Now.Subtract(DOB.Value).TotalDays / 365.25);
}
}
public Address PermanentAddress { get; set; }
public Address LocalAddress { get; set; }
}
}
Address.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
namespace KnockoutJSAPI.Models
{
public class Address
{
public string Street { get; set; }
public string City { get; set; }
public string State { get; set; }
public string PostalCode { get; set; }
public string Country { get; set; }
public string FullAddress
{
get
{
return string.Join(", ", this.GetType()
.GetProperties().Except(new List<PropertyInfo>() { this.GetType().GetProperty("FullAddress") }).Select(x => x.GetValue(this)));
}
}
}
}
Subject.cs
using System;
namespace KnockoutJSAPI.Models
{
public class Subject
{
public int SubjectId { get; set; }
public string SubjectName { get; set; }
public string Description { get; set; }
public int Credits { get; set; }
public string TeacherName { get; set; }
}
}
Student.cs
using System;
using System.Collections.Generic;
namespace KnockoutJSAPI.Models
{
public class Student : Person
{
public int StudentId { get; set; }
public int RollNo { get; set; }
public int ClassName { get; set; }
public char Section { get; set; }
public DateTime EnrollmentDate { get; set; }
public ICollection<Subject> Subjects { get; set; }
}
}
Set Up Routing: Ensure your Web API routing is correctly configured in
WebApiConfig.cs
.
using Newtonsoft.Json.Serialization;
using System.Web.Http;
namespace KnockoutJSAPI
{
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Web API configuration and services
// Configure JSON serialization to use snake_case
config.Formatters.JsonFormatter.SerializerSettings.ContractResolver =
new DefaultContractResolver { NamingStrategy = new SnakeCaseNamingStrategy() };
// Web API routes
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
}
Now create index.cshtml
and copy and paste this code into this file.
<section>
<!-- Display data if available -->
<div class="row g-3 row-cols-1 row-cols-md-2 row-cols-lg-3 row-cols-xl-4"
data-bind="foreach: items">
<!-- Column for each item -->
<div class="col">
<!-- Card to display item details -->
<div class="card ">
<!-- Item Image -->
<img data-bind="if: (photo), attr: { src: photo }" class="card-img-top" height="250">
<div class="card-body">
<!-- Card title bound to item's title -->
<h5 class="card-title text-capitalize" data-bind="text: full_name"></h5>
<!-- Card text bound to item's body -->
<p class="card-text">
<span data-bind="text: father_name"></span>,
<span data-bind="text: mother_name"></span>
</p>
</div>
<div class="card-footer border-0 pt-0">
<!-- Footer with item address -->
<p class="m-0 d-flex justify-content-between">
<span data-bind="text: permanent_address.full_address"></span>
</p>
</div>
</div>
</div>
</div>
</section>
<script type="text/javascript">
// Define the ViewModel
function AppViewModel() {
var self = this;
// Observable array to hold items
self.items = ko.observableArray([]);
// Function to load items from API
self.loadItems = function () {
$.getJSON('/api/Values', function (data) {
self.items(data); // Update items with data from API
console.log(data); // Log data to console for debugging
});
};
// Load items when ViewModel is instantiated
self.loadItems();
}
// Apply bindings to the ViewModel
ko.applyBindings(new AppViewModel());
</script>
And knockout.JS
CDN was added to in layout page like that,
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width" />
<title>@ViewBag.Title</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH" crossorigin="anonymous">
<!-- Link to Knockout.js library for MVVM pattern support -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.5.1/knockout-latest.min.js"
integrity="sha512-vs7+jbztHoMto5Yd/yinM4/y2DOkPLt0fATcN+j+G4ANY2z4faIzZIOMkpBmWdcxt+596FemCh9M18NUJTZwvw=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</head>
Output -
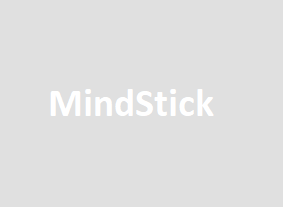
Leave Comment