Encrypting data in Knockout.js typically involves using JavaScript libraries for encryption combined with Knockout's data-binding capabilities. However, it's important to note that encryption should primarily be handled on the server side for security reasons. Client-side encryption can be used for additional security measures, such as encrypting data before sending it over the network.
Here’s a basic approach to implementing encryption in Knockout.js:
Using a JavaScript Encryption Library
Choose an Encryption Library: Select a JavaScript library that supports encryption algorithms, such as
CryptoJS
or sjcl
(Stanford JavaScript Crypto Library).
Include the Library: Add the library to your project by including it in your HTML file or through a module bundler like Webpack.
<script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/4.1.1/crypto-js.min.js"></script>
Encrypt Data in ViewModel: Use the library to encrypt data within your Knockout ViewModel. Here’s an example using
CryptoJS
:
function MyViewModel() {
var self = this;
self.secretKey = "hs66ahfa3928759823475928743kjashfkjas23465982345897234kzxcvz,cbv,zc823475923847"; // Replace with your secret key
self.plainText = ko.observable("");
self.encryptedText = ko.observable("");
self.encryptData = function() {
if(self.plainText()){
var encrypted = CryptoJS.AES.encrypt(self.plainText(), self.secretKey).toString();
self.encryptedText(encrypted);
}
};
self.decryptData = function() {
if(self.encryptedText()){
var decrypted = CryptoJS.AES.decrypt(self.encryptedText(), self.secretKey).toString(CryptoJS.enc.Utf8);
self.plainText(decrypted);
}
};
}
ko.applyBindings(new MyViewModel());
Binding in HTML: Bind your ViewModel functions to UI elements using Knockout’s data-bind attributes:
<section class="container">
<h1>Knockout.js Encryption Example</h1>
<hr/>
<div>
<input class="form-control" type="text" data-bind="value: plainText" placeholder="Enter text to encrypt" />
<button class="btn btn-primary mt-3" data-bind="click: encryptData">Encrypt</button>
</div>
<hr/>
<div>
<textarea class="form-control" data-bind="value: encryptedText" rows="4" cols="50"></textarea>
<button class="btn btn-secondary mt-3" data-bind="click: decryptData">Decrypt</button>
</div>
</section>
Notes:
- Security: Ensure your encryption keys are kept secure and not hard-coded in the client-side code.
- Server-side Encryption: For sensitive data, always consider server-side encryption and transmission over HTTPS.
- Compatibility: Test encryption libraries across browsers and ensure compatibility with your application’s environment.
Now bind the whole code,
<!DOCTYPE html>
<html>
<head>
<title>Knockout.js Encryption Example</title>
<!-- Link to Bootstrap CSS for styling -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH" crossorigin="anonymous">
<!-- Link to Knockout.js library for MVVM pattern support -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.5.1/knockout-latest.min.js"
integrity="sha512-vs7+jbztHoMto5Yd/yinM4/y2DOkPLt0fATcN+j+G4ANY2z4faIzZIOMkpBmWdcxt+596FemCh9M18NUJTZwvw=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/4.1.1/crypto-js.min.js"></script>
</head>
<body>
<section class="container">
<h1>Knockout.js Encryption Example</h1>
<hr/>
<div>
<input class="form-control" type="text" data-bind="value: plainText" placeholder="Enter text to encrypt" />
<button class="btn btn-primary mt-3" data-bind="click: encryptData">Encrypt</button> </div> <hr/> <div> <textarea class="form-control" data-bind="value: encryptedText" rows="4" cols="50"></textarea> <button class="btn btn-secondary mt-3" data-bind="click: decryptData">Decrypt</button> </div> </section> <script> function MyViewModel() { var self = this; self.secretKey = "hs66ahfa3928759823475928743kjashfkjas23465982345897234kzxcvz,cbv,zc823475923847"; // Replace with your secret key self.plainText = ko.observable(""); self.encryptedText = ko.observable(""); self.encryptData = function() { if(self.plainText()){ var encrypted = CryptoJS.AES.encrypt(self.plainText(), self.secretKey).toString(); self.encryptedText(encrypted); } }; self.decryptData = function() { if(self.encryptedText()){ var decrypted = CryptoJS.AES.decrypt(self.encryptedText(), self.secretKey).toString(CryptoJS.enc.Utf8); self.plainText(decrypted); } }; } ko.applyBindings(new MyViewModel()); </script> </body> </html>
Output -
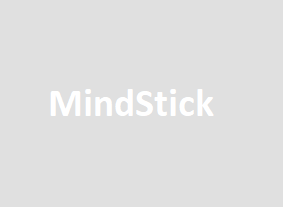
Why need it in knockout
Encryption is essential for ensuring the security and privacy of data, both at rest and in transit. Here are some key reasons why encryption is necessary:
1. Confidentiality: Encryption ensures that only authorized parties can access and understand the data. By encrypting sensitive information, even if unauthorized individuals gain access to the data, they cannot interpret it without the decryption key.
2. Data Integrity: Encryption helps maintain the integrity of data by protecting it from unauthorized modification. Encrypted data, when tampered with, becomes unreadable or the tampering becomes evident, thus alerting the rightful users to unauthorized access.
3. Compliance Requirements: Many industries and jurisdictions have legal and regulatory requirements mandating the protection of certain types of data through encryption. Adhering to these requirements helps organizations avoid penalties and maintain trust with customers and stakeholders.
4. Protection Against Data Breaches: In the event of a data breach or unauthorized access, encrypted data remains secure and unreadable without the decryption key. This mitigates the risk of sensitive information being exposed and exploited by malicious actors.
5. Securing Communication Channels: Encrypting data in transit (e.g., over the internet) protects it from interception and eavesdropping. Secure communication protocols such as HTTPS use encryption to ensure that data exchanged between clients and servers cannot be intercepted and read by unauthorized parties.
6. Maintaining Trust and Reputation: Implementing strong encryption measures demonstrates a commitment to protecting users' privacy and sensitive information. This helps build trust with customers, clients, and stakeholders who rely on the organization to safeguard their data.
7. Securing Client-Side Applications: In client-side applications like web browsers or mobile apps, encryption can add an extra layer of security, especially when handling sensitive user data locally or when transmitting data to servers.
8. Regulation and Standards Compliance: Industries such as finance, healthcare, and government have specific regulations (e.g., GDPR, HIPAA) that require encryption as part of data protection strategies. Compliance with these regulations is crucial for avoiding legal repercussions and maintaining operational continuity.
9. Protecting Personal and Financial Information: Encryption is particularly crucial for protecting personal identifiable information (PII) and financial data (e.g., credit card numbers, and banking details) from identity theft, fraud, and other malicious activities.
Leave Comment