Python loops are the constructs that allow working with a chunk of code by repeating its execution. It helps to eliminate the duplication process and increase the effectiveness of calculations or repeated actions in a particular situation. Python primarily supports two types of loops: for and while loops.
This is basically a first article and tries to explain the basic idea of Python loops as well as real-world uses of such loops. Loops—for and while—are crucial in repetitive tasks, which makes those constructs invaluable for most developers. It contains tips as well as additional sources for further study on the topic of the post.
Why Use Loops in Python?
Loops are vital for:
- Processing data sets.
- Simplify a complex process: self-performance and avoiding doing repetitive actions. Coordinate multiple steps in an operation.
- The use of procedures that call for more than a single loop.
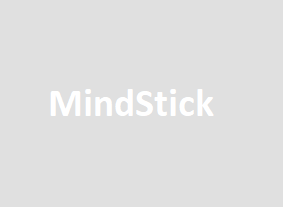
Types of Loops in Python
For Loop
A for loop goes through a sequence, which may be a list, tuple, dictionary, or range of values. It is especially useful for situations when the number of iterations is known beforehand. For instance, in cases of performing operations for every record in a database or names in a list.
Applications:
- Business analytics data collection processing, such as in banking and selling.
- This is because in order to iterate through the contents of the files for analysis, the files must be opened in the current session.
- Automating report generation.
While Loop
The while loop executes while a certain condition exists and retains its true value. It is most effective when used with data sets whose number of iterations cannot be predetermined, for instance, waiting for a user’s response or analyzing a connection to a network.
Applications:
- Real-time monitoring systems.
- Automating tasks adopting the time-based task model.
- Calculation procedures are evaluated employing dynamic algorithms.
The primary elements of a loop exist: The for-loop’s main components
1. Initialization
Operations may start with conditioning parameters for the loop or defining the range of a specific loop. For example, setting a counter in a while loop.
2. Condition
Every loop is dependent on a condition. With while loops, it is a logical expression, and with for loops, it is the sequence through which to iterate.
3. Iteration
Every loop proceeds through the block of code and, if necessary, changes a variable or gets the next value in its list.
4. Termination
The termination of a loop is based on the condition used in the named structure; as long as this condition is valid, the loop continues in its execution. One area of special attention is terminating techniques to avoid having endless recursion and iteration loops.
4. Real-World Scenarios
Loops are used in different areas of study. Here’s how they excel in real-life situations:
4.1 Data Processing
Loops are one of the most popular Python’s tools to manipulate data. For example:
- Scrolling through numerous fields within every row.
- Sweeping activities such as filling the missing values can also be automated.
- Summarizing statistics.
There are other libraries available for the looping efficiency of the data, such as Pandas and NumPy for Python.
4.2 Automation Tasks
Loops automate mundane and repetitive processes.
- A mode of emailing that involves communicating with postings of significant quantity to numerous individuals at a given time.
- Data scraping using web scraping tools such as BeautifulSoup.
- Restructurating the cabinet’s shelves or drawers and renaming their folders.
Check out this simple browser project for an idea on how to go about it.
4.3 Game Development
Loops guarantee the game’s rendering and updating (mostly at the screen-refresh rate). These involve:
- Processing of events (keyboard and mouse).
- Managing character movement.
- Changing screen graphic displays after some time.
4.4 Machine Learning
In AI and ML, loops iterate through datasets to:
- Train models in batches.
- Verify the measure variables for the purposes of assessing the performance.
- Fine-tune hyperparameters.
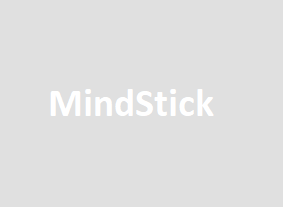
5. Best Practices
- Avoid Infinite Loops: It is very important that loop conditions are clearly stated.
- Use Enumerate for Indexing: A neater way of referring to indices in sequences.
- Leverage Comprehensions: Eliminate all the uses of loops wherever they are present and replace them with list or dictionary comprehension appropriately.
Suggestions for Employing Python Loops
- Avoid Infinite Loops: As a programmer, always make sure that your loop conditions are clear, accurate, and specific; otherwise, unexpected things may happen.
- Use Enumerators and Itertools: This is due to the fact that Python provides enhanced tools like enumerate() for the iteration processes.
- Optimize Performance: In specific cases where data handling is concerned but the volume is inherently big, then avoid nesting of loops or go for generator expressions.
Conclusion
For loops are probably one of the most important building blocks for Python programming, giving increased flexibility and functionality for real-world scenarios. From handling data to automating processes, knowing your way around Python loops helps towards being a better developer. Begin practicing Python loops today to explore the idea of applying any type of challenge that may come across.
Leave Comment