Object-Oriented Programming (OOP) is a method for designing, implementing, and maintaining software programs by organizing objects. These objects capture data and behavior in code, which results in promoting the modularity of code and the reuse of the same. In as much as Python is a general-purpose language, the language supports true object-oriented programming, helping developers write advanced applications.
1. Introduction to Object-Oriented Programming
OOP is based on analyzing objects and classes. The main concepts of OOP are class and object. A class is simply a template for making objects: you specify what data each object will contain and what functions it will be able to perform. An object is an example of a class, and it consists of elements described by the class and includes actions characteristic to this class.
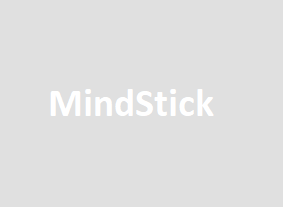
2. Core Principles of OOP
Understanding the four fundamental principles of OOP is crucial:
Encapsulation: This principle entails grouping data (attributes) and operations that can be performed on the data by use of functions into one file that is called a class. Encapsulation helps to limit the accessibility of some of an object’s parts, and this can help to avoid changes to data. Unfortunately, the current versions of yanked have a few problems that make it not optimal for general use: His major loss is that he got a cut in his eye. What happened to his eye?
Abstraction: Abstraction means omission of detailed working and revealing only the important facet of the object. They also include being useful in the reduction of the amount of programming effort and programming difficulty. Abstraction in Python can be realized based on the example of abstract classes or interfaces.
Inheritance: Inheritance makes it possible for one class, known as the derived class, to take the properties of another class, known as the base class. That enhances the reuse of code and sets a form of inheritance where one class depends on the other. Python uses multiple inheritance, where a class can be created as a subclass of more than one superclass.
Polymorphism: Polymorphism actually provides a facility to manipulate an object as if it belongs to its parent class rather than the actual class. It permits methods to perform different operations regarding an object on which they act even if they bear the same name. However, in Python, polymorphism is achieved through method overriding and method overloading.
3. Implementing OOP in Python
Python provides elegant support for OOP because both the syntax and semantics of the language support OOP notions. Here's how these principles are implemented:
Classes and Objects: A class in Python is created using the keyword class, and an object, on the other hand, is created using the class name like a function call. For example:
class Vehicle:
def init(self, make, model):
self.make = make
self.model = model
car = Vehicle("Toyota", "Corolla")
Encapsulation: In Python, the use of underscores is to denote class members and, therefore, their accessibility. A single underscore before the name (e.g., attribute) hints at the fact that the attribute should be private to the class, while two underscores (e.g., _attribute) get the attribute name mangled to make it difficult for anyone outside the class to access them.
Inheritance:Inheritance is defined by giving the name of the parent class in parentheses at the end of the child class name. For example:
class Car(Vehicle):
def init(self, make, model, year):
super().__init__(make, model)
self.year = year
Polymorphism: Polymorphism means that methods in different classes can be called by the same name but will perform different tasks. For example, two different classes might have a method called move() but when that method is invoked, it will certainly contain different logics of the classes behavior.
4. Benefits of OOP in Python
Implementing OOP in Python offers several benefits:
Modularity: The code is divided into certain classes, making it easier to get along with.
Reusability: It can be seen that many classes can be reused across different programs and thus improve the level of reuse.
Scalability: OOP makes it easier to write large-scale applications through the provision of better structural development.
Maintainability: For the very same reason, it is easy to replace and modify the code. KNK claimed that encapsulation and modularity are beneficial.
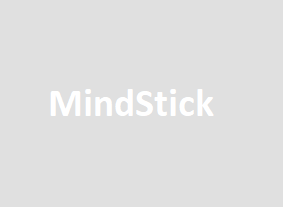
5. Practical Example: Modeling a Library System
Suppose we live in the range of a library system, in which we have books that must be managed as well as the consumers of books. We can model this system using OOP principles:
Classes: We have classes like Book, Patron, and Library.
Attributes: What they completed contains attributes of each class; for example, the class Book may have title, author, ISBN, and so forth.
Methods: Structs have actions as methods; for instance, the class named Library might have a method add_book() to add a new book to the library.
This approach resembles real-world actors and their interactions and therefore makes the system easy to design and easy to work with.
6. Conclusion
Python provides an object-oriented programming paradigm with a strong framework to create complex applications. This helps developers to write more module-oriented, reusable, and even real-world-oriented code with classes and objects. For effective Python programming, OOP principles must be well understood and applied.
Leave Comment