In this article i am going to demonstrate how to upload , drag and drop file in asp.net. here i am using ajax and handler to upload drop file to the server.
Step 1
Open visual studio create a website and give the suitable name.Step 2
Add new web formStep 3
Put below script on the head of page<script src="//code.jquery.com/jquery-1.12.0.min.js"></script>
<script>
$(document).ready(function () {
var Divobj = $("#drgdiv");
Divobj.on('dragenter', function (e) {
$(this).css('border', '1px solid green');
e.stopPropagation();
e.preventDefault();
});
Divobj.on('dragover', function (e) {
e.stopPropagation();
e.preventDefault();
});
$(document).on('dragenter', function (e) {
e.stopPropagation();
e.preventDefault();
});
$(document).on('dragover', function (e) {
e.stopPropagation();
e.preventDefault();
obj.css('border', '1px dotted red');
});
$(document).on('drop', function (e) {
e.stopPropagation();
e.preventDefault();
});
Divobj.on('drop', function (e) {
$(this).css('border', '1px dotted red');
e.preventDefault();
var files = e.originalEvent.dataTransfer.files;
for (var i = 0; i < files.length; i++) {
var fd = new FormData();
fd.append('file', files[i]);
//==========Start Upload drop file to server using ajax and handler
var choice = {};
choice.url = "Upload.ashx";
choice.type = "POST";
choice.data = fd;
choice.contentType = false;
choice.processData = false;
choice.success = function (result) {
$("#upld").append("<li>" + result + "</li>");
};
choice.error = function (err) { //alert(err.statusText);
};
$.ajax(choice);
event.preventDefault();
//==========End Upload drop file to server using ajax and handler
}
});
});
</script>
Html Block
<div id="drgdiv" style="height: 200px; border: 1px solid teal; text-align:center; font-size:xx-large; padding-top:30px;">
Drag & drop file here
</div>
===== Uploaded File ======
<ul id="upld">
</ul>
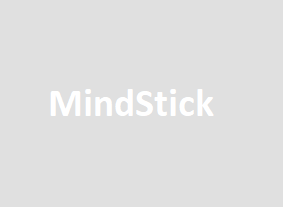
Step 4
Right click on solutions explorer Add new Item => Add generic handler
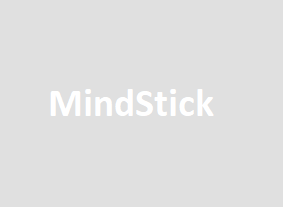
And give suitable name to the handler lets upload.ashx
Add below code snippet
using System;
using System.Web;
using System.IO;
using System.Drawing;
using System.Drawing.Drawing2D ;
public class Upload : IHttpHandler {
public void ProcessRequest (HttpContext context) {
string returnPath = "";
if (context.Request.Files.Count > 0)
{
HttpFileCollection SelectedFiles = context.Request.Files;
for (int i = 0; i < SelectedFiles.Count; i++)
{
HttpPostedFile PostedFile = SelectedFiles[i];
string generatedFilename = PostedFile.FileName;
string FileNameBig = context.Server.MapPath("~/Upload/")+ generatedFilename;
PostedFile.SaveAs(FileNameBig);
returnPath = PostedFile.FileName;
}
}
else
{
context.Response.ContentType = "text/plain";
context.Response.Write("Please Select Files");
}
context.Response.ContentType = "text/plain";
context.Response.Write(returnPath);
}
public bool IsReusable {
get {
return false;
}
}
}
Results
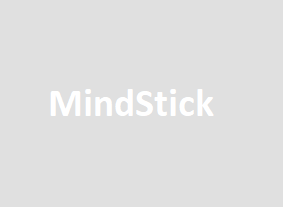
Anonymous User
20-Feb-2016John Smith
20-Feb-2016