Client-side form validation in JavaScript can be performed by adding event listeners to form elements and using JavaScript to check the values of form fields before submission. This can improve user experience by providing immediate feedback and reducing the load on the server.
Here's a step-by-step guide on how to perform client-side form validation:
Basic Steps for Form Validation
- Get References to Form Elements: Use JavaScript to reference the form and its elements.
- Add Event Listeners: Attach event listeners to the form and its elements to handle user input and form submission.
- Validate Input: Write validation functions to check the values of form fields.
- Provide Feedback: Show error messages or feedback to the user based on validation results.
Example: Form Validation
HTML Form
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Bootstrap demo</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH" crossorigin="anonymous">
<style type="text/css">
:root,
*{
font-size: large;
}
form label.star,
form label.required {
content: '';
font-weight: 500;
}
form label.star::after,
form label.required::after {
margin-left: 10px;
content: '*';
color: red;
}
input[type='password'] {
letter-spacing: 2px;
}
.input-error {
color: red;
font-size: 0.9em;
font-weight: 500;
margin: 0.5rem auto;
}
</style>
</head>
<body>
<div class="container">
<div class="my-4">
<form id="myForm" class="row g-3" >
<div class="col-12">
<label for="username" class="form-label fw-bold star">
Username
</label>
<input class="form-control" placeholder="User Name" type="text" id="username" name="username" />
<span id="usernameError" class="input-error"></span>
</div>
<div class="col-12">
<label for="email" class="form-label fw-bold star">Email</label>
<input class="form-control" placeholder="Email" type="text" id="email" name="email" />
<span id="emailError" class="input-error"></span>
</div>
<div class="col-12">
<label for="password" class="form-label fw-bold star">Password </label>
<input class="form-control" placeholder="********" type="password" id="password" name="password" />
<span id="passwordError" class="input-error"></span>
</div>
<div class="col-12">
<button class="btn btn-primary" type="submit">Submit</button>
</div>
</form>
</div>
</div>
</body>
</html>
CSS For Error message
.input-error {
color: red;
font-size: 0.9em;
font-weight: 500;
margin: 0.5rem auto;
}
JavaScript for form validation
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', function() {
var formData = null;
const form = document.getElementById('myForm');
const username = document.getElementById('username');
const email = document.getElementById('email');
const password = document.getElementById('password');
const usernameError = document.getElementById('usernameError');
const emailError = document.getElementById('emailError');
const passwordError = document.getElementById('passwordError');
form.addEventListener('submit', function(event) {
// Clear previous error messages
usernameError.textContent = '';
emailError.textContent = '';
passwordError.textContent = '';
// Validate each field
let isValid = true;
if (username.value.trim().length < 3) {
usernameError.textContent = 'Username must be at least 3 characters long.';
isValid = false;
username.classList.add("is-invalid");
} else {
// Clear any previous error message
usernameError.textContent = '';
// Remove the "is-invalid" class if username is valid
username.classList.remove("is-invalid");
}
if (!validateEmail(email.value)) {
emailError.textContent = 'Please enter a valid email address. Like (xyx@gmail.com)';
isValid = false;
}
if (password.value.trim().length < 6) {
passwordError.textContent = 'Password must be at least 6 characters long.';
isValid = false;
}
if (!isValid) {
event.preventDefault(); // Prevent form submission if validation fails
}
});
// Function to validate email using a simple regex
function validateEmail(email) {
const re = /^[\w.-]+@[\w.-]+\.[\w]{2,}$/;
return re.test(email.toLowerCase());
}
});
Output :
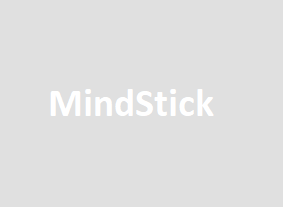
Explanation
HTML Form:
- The form includes fields for username, email, and password.
- Each field has a corresponding
<span>
element to display error messages.
CSS for Error Messages (optional):
- Error messages are styled in red to make them noticeable.
JavaScript for Validation:
- The JavaScript code is executed when the DOM content is loaded.
- References to form elements and error message containers are obtained using
getElementById
. - An event listener is added to the form to handle the
submit
event. - The
submit
event handler function validates the form fields:- It clears any previous error messages.
- It checks the length of the username and password, and validates the email format.
- If any validation fails, it sets the corresponding error message and prevents form submission by calling
event.preventDefault()
.
Benefits of Client-Side Validation
- Immediate Feedback: Users get immediate feedback on their input, which improves user experience.
- Reduced Server Load: Reduces unnecessary server requests by catching invalid inputs early.
- Enhanced User Experience: Provides a more responsive and interactive form experience.
Leave Comment