Angular js Custom Filter
In AngularJS, custom filters are used to set the value of an expression to be displayed to the user.
{{ expression | filterName:argument }}
Syntax, where filterName
is the name of the optional filter function.
Defining Custom Filters
Here are the way to define the custom filter in Angular js,
Using the filter
method
angular.module('myApp', [])
.filter('customFilter', function() {
return function(input, arg1, arg2) {
// Filter logic here
return /* transformed output */;
};
});
filter('customFilter', function() {...})
Defines a filter named customFilter.return function(input, arg1, arg2) { ... }
The middle function is a filter function that acceptsinput
(the value to be selected) and any other arguments (arg1
,arg2
).
Injecting dependencies
If you need custom filter dependencies (e.g., other functions or utilities), add them to the filter function as you would for any AngularJS component.
Custom Filters
Once defined, you can apply custom filters to your HTML template.
<div ng-controller="MyController">
<p>{{ someText | customFilter:arg1:arg2 }}</p>
</div>
someText
Text to change.
customFilter
The name of a predefined filter.
arg1
, arg2
Optional text passed to the filter function.
Example-
Here is a simple Angular js example to demonstrates the custom filter,
<!DOCTYPE html>
<html data-ng-app="myApp">
<head>
<meta charset='utf-8'>
<meta http-equiv='X-UA-Compatible' content='IE=edge'>
<title>ng-Root Scope</title>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<!-- bootstrap cdn -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- angular js cdn -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
</head>
<body data-ng-controller ="myController">
<div class="container my-4">
<h3>Text transform into lower case using custom filter</h3>
<table class="table my-2">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Name</th>
</tr>
</thead>
<!-- bind the data from array with filter and orderBy -->
<tbody data-ng-repeat="name in names | orderBy : name">
<tr>
<th scope="row">{{$index + 1}}</th>
<!-- add custom filter here -->
<td data-ng-bind="name | customFilter"></td>
</tr>
</tbody>
</table>
</div>
<script>
angular.module("myApp", [])
.controller("myController", ['$scope', function($scope){
// Array data
$scope.names = [ "Jani", "Carl", "Margareth", "Hege", "Doe", "Gustav", "Birgit", "Mary", "Kai" ];
}])
// create custom filter that return each value in lower case
.filter('customFilter', function(){
return function(name){
return name.toLowerCase();
}
});
</script>
</body>
</html>
In the example above-
$scope.names
is the array of data.customFilter
is the name of the created custom filter.- Converted text to lower-case using the JavaScript
toLowerCase(
) method.
Output-
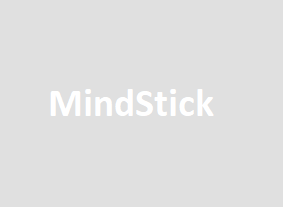
Also, Read: What is $rootScope in AngularJS?
Leave Comment